
Syntax introduced:
[ ] ' ( ) find linspace zeros ones eye sqrt length size reshape diag plot fplot line hold on/off axis
Make a 1D vector and query its attributes
You can create a row vector by naming each of its elements explicitly. Put them inside square brackets with commas or spaces separating elements. For example, I'm going to create a row vector and name it myVector. It's going to have 5 numbers in it.
>> myVector = [1, 75, 0.3, 1000, 2^4] myVector = 1.0e+03 * 0.0010 0.0750 0.0003 1.0000 0.0160
Okay, that's fine. Let's say I already forgot what the fourth number in myVector is. Find out by asking MATLAB. Use parentheses to specify that you want to know the value of the 4th entry:
>> myVector(4) ans = 1000
MATLAB will give you an error message if you try to ask it for a value that doesn't exist:
>> myVector(6) Index exceeds matrix dimensions.In the example above, myVector only has 5 numbers in it, so asking for the value of the sixth number doesn't make sense. The command size is useful for reminding yourself about the dimensions of a vector or matrix. When you ask MATLAB for the size of a vector or matrix it returns a vector with two entries: the number of rows and the number of columns.
>> size(myVector) ans = 1 5
Logical operators
The command find is good if you want to search for specific entries:
>> find(myVector==1000) ans = 4 >> find(myVector>1) ans = 2 4 5
In the above examples using find, MATLAB returns the indices (positions) of the values of the vector that match what you were looking for, not the values themselves. When I wrote find(myVector==1000) I was asking MATLAB which number in myVector is equal to 1000. MATLAB answered that it was the 4th entry. When I wrote find(myVector>1) I was asking MATLAB which numbers in myVector have values greater than 1. MATLAB answered that the 2nd, 4th, and 5th entries are greater than 1.
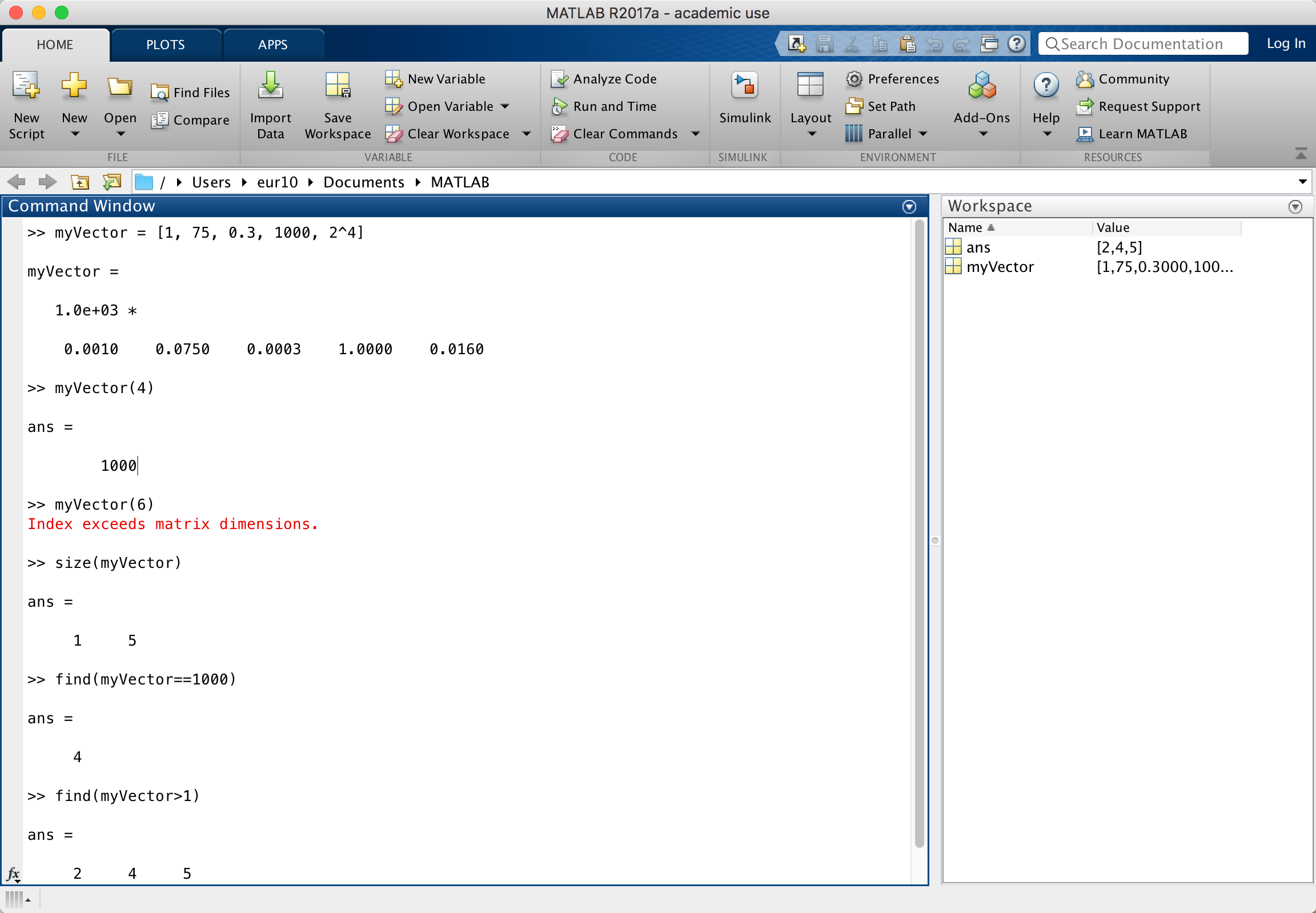
The difference between = and ==
One = is an assignment. It is an action. It means I am setting something equal to something else. For example: x = 3 means I am declaring that there is a variable x and its value is 3. y = [1 2 3 4 5] means I am declaring a variable y and it is a 1x5 vector whose values are 1, 2, 3, 4, and 5. If I write z=y then I've made a copy of y and called it z.
Two == is a logical test, not an assignment. It is essentially asking whether something equals something else. When I wrote find(myVector==1000) I was asking MATLAB to tell me the position in myVector whose value equals 1000.
Other common logical operators are ~ (not) > greater than < less than. Logical operators can be combined as in find(myVector>=1) which would find all the positions in myVector whose values are greater than or equal to 1.